创建vue3项目
大约 4 分钟
创建Vue3项目
Vite 构建项目
Vite(法语意为 "快速的",发音 /vit/
发音同 "veet")是一种新型前端构建工具,能够显著提升前端开发体验。它主要由两部分组成:
- 一个开发服务器,它基于 原生 ES 模块 提供了 丰富的内建功能,如速度快到惊人的 模块热更新(HMR)。
- 一套构建指令,它使用 Rollup 打包你的代码,并且它是预配置的,可输出用于生产环境的高度优化过的静态资源。
Vite 意在提供开箱即用的配置,同时它的 插件 API 和 JavaScript API 带来了高度的可扩展性,并有完整的类型支持。
运作方式
Vite 以 原生 ESM 方式提供源码。这实际上是让浏览器接管了打包程序的部分工作:Vite 只需要在浏览器请求源码时进行转换并按需提供源码。根据情景动态导入代码,即只在当前屏幕上实际使用时才会被处理。
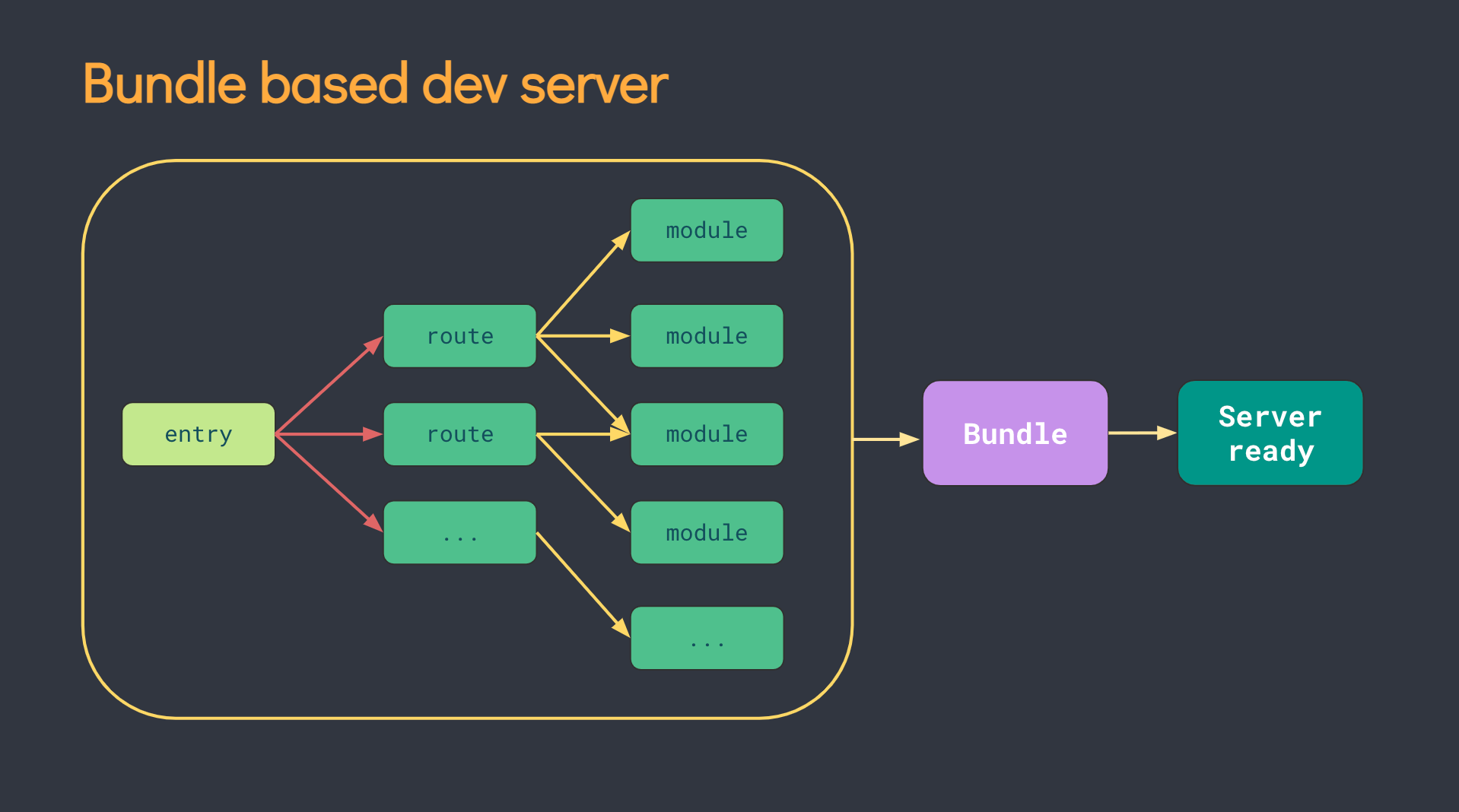
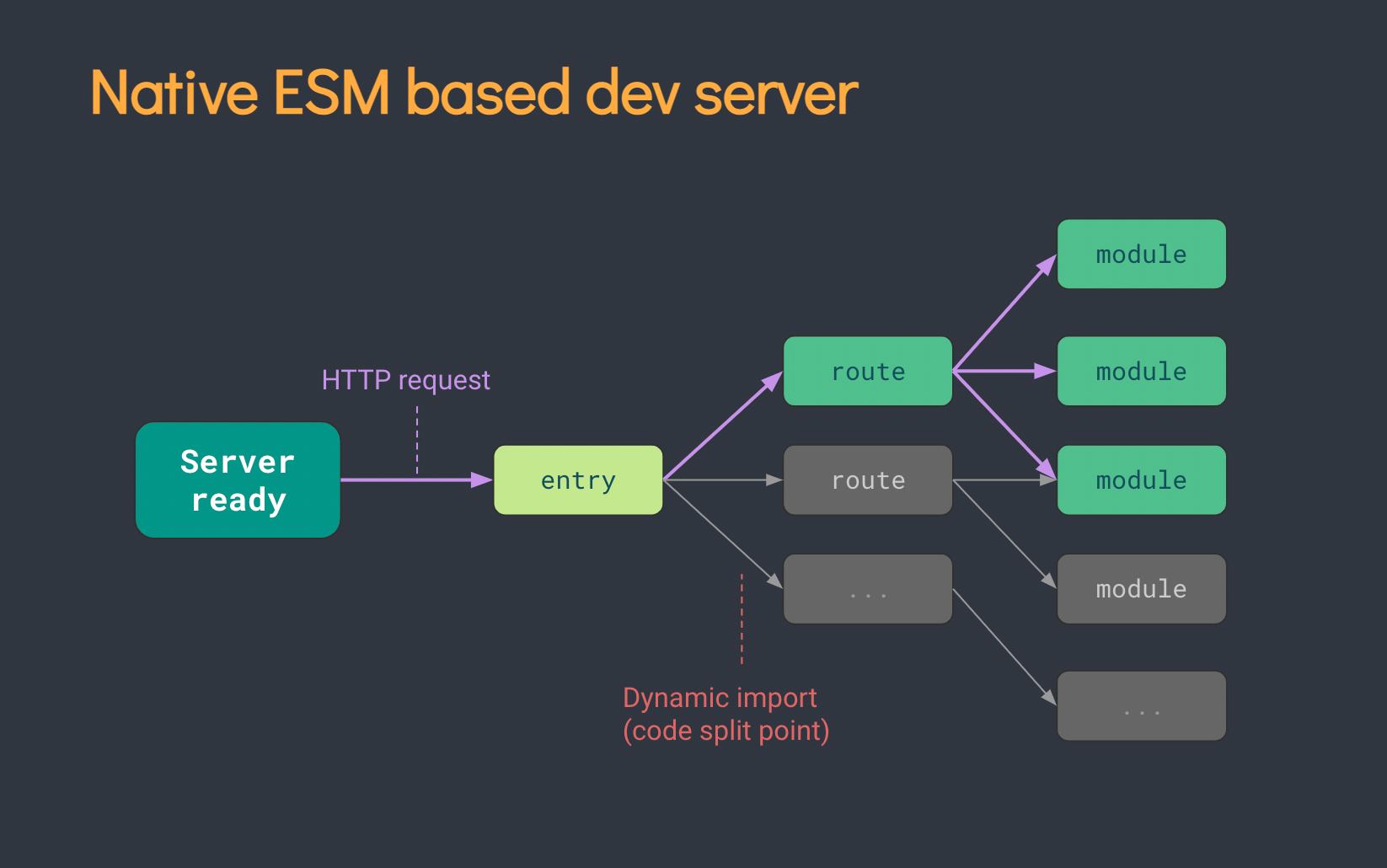
安装 & 启动
兼容性注意
Vite 需要 Node.js 版本 14.18+,16+。然而,有些模板需要依赖更高的 Node 版本才能正常运行,当你的包管理器发出警告时,请注意升级你的 Node 版本。
不使用模板 【推荐】
npm create vite@latest
yarn create vite
pnpm create vite
Project name: 填写项目名
Select a framework: 选择一个框架
Select a variant: 选择开发语言
前往创建好的项目执行
yarn
安装所有依赖启动项目 可在
package.json
中设置启动项目和打包项目的命令
"scripts": {
"dev": "vite",
"build": "vue-tsc --noEmit && vite build",
"preview": "vite preview"
},
关闭严格模式(强迫症患者)
如果代码报红,你又有强迫症,那你应该去
tsconfig.json
中设置 "strict": false, 然后重启VsCode
vscode 插件推荐
下载Vue Language Features (Volar)
下载TypeScript Vue Plugin (Volar)
如果下载了 vetur需要卸载掉vetur
Vite.config.js基本配置
import { defineConfig } from 'vite'
import vue from '@vitejs/plugin-vue'
// 导入内置对象path 如果不能识别path 请检查是否安装了@types/node,需要安装 yarn add @types/node
import path from "path"
// https://vitejs.dev/config/
export default defineConfig({
// 插件
plugins: [vue()],
// 各种别名
resolve:{
// 给项目中的各种文件夹取别名
alias:{
'@':path.resolve(__dirname,'./src')
}
},
// css预加载配置
css:{
preprocessorOptions:{
// style中的lang为scss时自动加载以下内容
scss:{
additionalData:`@import '@/style/var.scss';@import '@/style/mimix.scss';`,
}
}
}
})
安装sass
npm i sass -D
yarn add sass -D
pnpm i sass -D
配置router配置
1.安装vue-router(4.x版本)
yarn add vue-router
2.创建文件:src/router/index.ts
创建文件:src/router/index.ts
- 创建router并导出
- 配置routes
// createRouter是一个函数,执行之后返回一个router对象(类比vue2:new一个router),函数式编程
import { createRouter, createWebHashHistory, RouteRecordRaw } from 'vue-router'
const routes: RouteRecordRaw[] = [
// 重定向到/login
{
path:'/',
redirect:'/login',
},
//登录页
{
path: '/login',
component: () => import('@/views/login.vue'),
},
//首页
{
path: '/index',
component: () => import('@/views/index.vue')
}
]
// 调用createRouter,得到一个router对象
const router = createRouter({
// 理解为vue2里面的那个model(路由的模式: hash)
history: createWebHashHistory(),
routes
})
// 导出之后跟vue应用联系在一起
export default router
3.main.ts 中导入router
import { createApp } from 'vue'
import './style.css'
import App from './App.vue'
import router from './router'
const app = createApp(App)
// 注入router
app.use(router)
app.mount('#app')
Composition (组合)API VS Option(配置型) API
- 配置项 Option API 数据,方法,计算属性等等 写在规定的配置项里面
- Composition API 组合式API
- 我们可以更加优雅的组织我们的代码,函数。让相关功能的代码更加有序的组织在一起
- 配置项API vs 组合式API