Flutter弹窗
大约 4 分钟
官网地址:选择你的开发平台,开始使用 | Flutter 中文文档 - Flutter 中文开发者网站 - Flutter
文档地址:第二版序 | 《Flutter实战·第二版》 (flutterchina.club)
FlutterDialog
1、AlertDialog(普通弹出框)
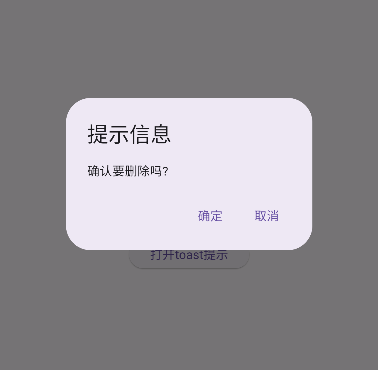
1.1、抽离AlertDialog.dart
通过var res = await showDialog(...)获取Navigator.of(context).pop('确定')点击后在pop中获取值
import 'package:flutter/material.dart';
//alert弹窗
alertDialog(
dynamic context, {
String title = "提示",
String content = "内容",
String confirmText = "确定",
String cancelText = "取消",
bool barrierDismissible = false,
Function()? confirm,
Function()? cancel,
}) async {
var res = await showDialog(
barrierDismissible: barrierDismissible, //表示点击灰色背景的时候是否消失弹出框
context: context,
builder: (context) {
return AlertDialog(
title: Text(title), //标题
content: Text(content), //内容
actions: [
//下方动作
TextButton(onPressed: confirm, child: Text(confirmText)),
TextButton(
onPressed: cancel ?? //如果传了cancel就使用传入的函数否则使用后面的迷人取消
() {
Navigator.of(context).pop('点击了取消');
},
child: Text(cancelText))
],
);
});
return res;
}
1.2、导入AlertDialog.dart并且使用
import 'package:flutter/material.dart';
// 导入alert弹窗
import './alertDialog.dart';
//按钮点击调用
ElevatedButton(
onPressed: () async {
var res = await alertDialog(
context,
title: '提示信息',
content: '确认要删除吗?',
confirm: () {
print('点击了确认');
Navigator.of(context).pop('关闭');
},
);
print(res);
},
child: const Text('打开AlertDialog'))
2、SimpleDialog和SimpleDialogOption(选择弹出框)
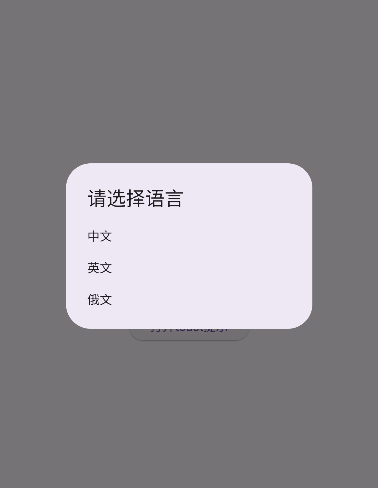
2.1、抽离AlertDialog.dart
import 'package:flutter/material.dart';
// SimpleDialog弹窗
simpleDialog(
dynamic context, {
String title = "标题",
bool barrierDismissible = false,
}) async {
var res = await showDialog(
barrierDismissible: barrierDismissible, //表示点击灰色背景的时候是否消失弹出框
context: context,
builder: (context) {
return SimpleDialog(title: Text(title), children: [
SimpleDialogOption(
child: const Text('中文'),
onPressed: () {
Navigator.pop(context, '你选择了中文');
}),
SimpleDialogOption(
child: const Text('英文'),
onPressed: () {
Navigator.pop(context, '你选择了英文');
}),
SimpleDialogOption(
child: const Text('俄文'),
onPressed: () {
Navigator.pop(context, '你选择了俄文');
}),
]);
});
return res;
}
2.2、导入AlertDialog.dart并且使用
import 'package:flutter/material.dart';
// 导入simpleDialog弹窗
import './simpleDialog.dart';
//点击调用
ElevatedButton(
onPressed: () async {
var res = await simpleDialog(context, title: '请选择语言');
print(res);
},
child: const Text('打开SimpleDialog')),
3、showModalBottomSheet底部弹出框
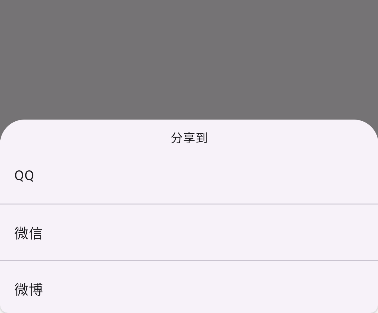
3.1、抽离showModalBottomSheet.dart
import 'package:flutter/material.dart';
// showModalBottomSheet弹窗
ShowBottomSheet(dynamic context) async {
var res = await showModalBottomSheet(
context: context,
builder: (context) {
return SizedBox(
height: 220,
child: Column(
children: <Widget>[
Container(
padding: const EdgeInsets.all(10),
child: const Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [Text('分享到')],
),
),
ListTile(
title: const Text("QQ"),
onTap: () {
Navigator.pop(context, "QQ");
},
),
const Divider(),
ListTile(
title: const Text("微信"),
onTap: () {
Navigator.pop(context, "微信");
},
),
const Divider(),
ListTile(
title: const Text("微博"),
onTap: () {
Navigator.pop(context, "微博");
},
)
],
),
);
});
print(res);
}
3.2、导入showModalBottomSheet.dart并且使用
import 'package:flutter/material.dart';
// 导入showModalBottomSheet弹窗
import './showModalBottomSheet.dart';
//点击调用
ElevatedButton(
onPressed: () async {
var res = await ShowBottomSheet(context);
print(res);
},
child: const Text('底部弹出框showModalBottomSheet')),
fluttertoast的使用
4.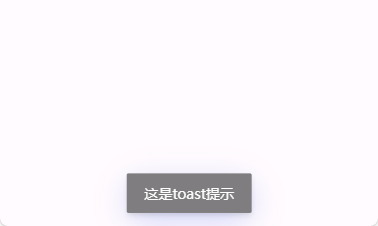
4.1、在pubspec.yaml中的dependencies:下添加
fluttertoast: ^8.0.9
4.2、抽离flutterToast.dart(封装成函数灵活调用,避免多处导入使用)
// 导入fluttertoast
import 'package:fluttertoast/fluttertoast.dart';
//showToast提示
showToast({
required String msg,
ToastGravity gravity = ToastGravity.BOTTOM,
int timeInSecForIosWeb = 1,
String webBgColor = "rgba(0,0,0,0.5)",
String webPosition = "center",
}) {
Fluttertoast.showToast(
msg: msg,
toastLength: Toast.LENGTH_SHORT,
gravity: gravity,
timeInSecForIosWeb: timeInSecForIosWeb, //时长
webPosition: webPosition, //出现位置
webBgColor: webBgColor, //背景颜色
);
}
4.3、导入flutterToast.dart并使用
import 'package:flutter/material.dart';
// 导入fluttertoast
import './flutterToast.dart';
//点击调用
ElevatedButton(
onPressed: () {
showToast(msg: '这是toast提示');
},
child: const Text('打开toast提示')),
ftoast的使用
5、支持LINUX MACOS WEB WINDOWS的另一个插件
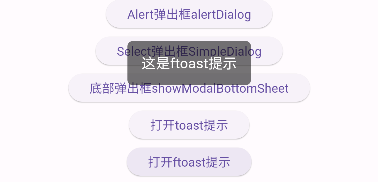
5.1、在pubspec.yaml中的dependencies:下添加
ftoast: ^2.0.0
5.2、单独抽离ftoast.dart
// ftoast
import 'package:ftoast/ftoast.dart';
//showfToast提示
showfToast(
context, {
String msg = "消息",
}) {
FToast.toast(
context,
msg: msg,
);
}
5.3、导入ftoast.dart使用
import 'package:flutter/material.dart';
// 导入showftoast
import './ftoast.dart';
//点击调用
ElevatedButton(
onPressed: () {
showfToast(context, msg: '这是ftoast提示');
},
child: const Text('打开ftoast提示')),
6、自定义Flutter Dialog 、Material组件、InkWell组件
自定义Dialog对象,需要继承Dialog类,尽管Dialog提供了child参数可以用来写视图界面,但是往往会
达不到我们想要的效果,因为默认的Dialog背景框是满屏的。如果我们想完全定义界面,就需要重写
build函数。
6.1、自定义一个提示的Dialog
6.1.1、新建myDialog.dart
//自定义MyDialog类和调用方法
import 'package:flutter/material.dart';
// 打开对话框函数
showMyDialog(
context, {
bool barrierDismissible = false,
String title = "标题",
String content = "内容",
Function()? onClosed,
}) async {
var res = await showDialog(
barrierDismissible: true, //表示点击灰色背景的时候是否消失弹出框
context: context,
builder: (context) {
return MyDialog(
title: title,
onClosed: onClosed ??
() {
Navigator.of(context).pop('点击了关闭');
},
content: content);
});
return res;
}
// 自定义MyDialog对话框内容
// ignore: must_be_immutable
class MyDialog extends Dialog {
String title;
String content;
Function()? onClosed;
MyDialog(
{Key? key,
required this.title,
required this.onClosed,
this.content = ""})
: super(key: key);
Widget build(BuildContext context) {
return Material(
type: MaterialType.transparency,
child: Center(//在这里可以定义你想要的样式
child: Container(
height: 300,
width: 300,
color: Colors.white,
child: Column(
children: <Widget>[
Padding(
padding: const EdgeInsets.all(10),
child: Stack(
children: <Widget>[
Align(
alignment: Alignment.center,
child: Text(title),
),
Align(
alignment: Alignment.centerRight,
child: InkWell(
onTap: onClosed,
child: const Icon(Icons.close),
),
)
],
),
),
const Divider(),
Container(
padding: const EdgeInsets.all(10),
width: double.infinity,
child: Text(content, textAlign: TextAlign.left),
)
],
),
)),
);
}
}
6.1.2、调用myDialog.dart
import 'package:flutter/material.dart';
// 导入自定义MyDialog对话框
import './myDialog.dart';
//点击调用
ElevatedButton(
onPressed: () async {
var res = showMyDialog(context, title: '标题', content: '内容',
onClosed: () {
Navigator.of(context).pop('关闭');
});
print(res);
},
child: const Text('打开自定义Dailog')),
7、定时器
定义一个定时器自动关闭上方的Dialog
7.1、使用定时器
//定时器需要使用async库
import 'dart:async';
//设置时间
const timeout = Duration(seconds: 3);
//创建定时器
var t=Timer.periodic(timeout, (timer) {
print('afterTimer='+DateTime.now().toString()););
// timer.cancel(); // 取消定时器
});
t.cancel(); // 取消定时器
7.2、记得要取消定时器
//销毁组件时取消
void dispose() {
super.dispose();
t.cancel();
}